A simple user defined function in R
- Andrew Jones
- Aug 2, 2017
- 2 min read
For our post on user-defined functions in Python - click here!
A function is essentially a block of re-usable code that takes inputs, performs a specific task, and subsequently provides an output when called.
You'll have seen these in action already, as R contains a number of built in functions, for example print(), sum(), and max()
However, in the case where we need to undertake a task specific to our needs, we can create a 'user-defined' function
Writing an R function can be broken down into the following seven parts
1. Function Name
This is what the function will be referred to as when we call the function later on
1. function
function is the R keyword that signals we're creating a function
3. Input Variables
The names of the variable inputs that will be processed by the function when we call it later on. These will be included within parenthesis, and if there are multiple inputs these need to be separated by a comma
4. { }
The braces are necessary syntax that surround the body of an R function
5. Function Body
The code specific to the task or calculation we want the function to undertake
6. return
Return is another R keyword, as in this case it specifies what part of the task or calculation we want to output
7. Calling the Function
We use the function name to call our specific function, and provide the inputs for the function to process. Running this will give us an output!
Example: In this scenario we need a function that can take a Celsius temperature and convert it to Fahrenheit for us.
Here is the code:
temp_converter <- function(celsius) { fahrenheit <- celsius*9/5 + 32 return(fahrenheit) }
We start by naming our function (temp_converter) then proceed to use the function keyword and in parenthesis we put the name of input variable(s) (celsius)
Within braces, we code in the calculation for Celsius to Fahrenheit conversion, and beneath this we specify that we want to return the value of Fahrenheit.
That's it - the function is complete!
Let's now call this function and see it in action.
First, specify the function name (temp converter) and then provide an input value for Celsius. The function will take this value and convert to Fahrenheit for us:
temp_coverter(0) [1] 32.0
temp_coverter(100) [1] 212.0
temp_coverter(35.5) [1] 95.6
It works!
Fun fact, there is one temperature where Celsius and Fahrenheit are the same...
temp_coverter(-40) [1] -40
Hope you enjoyed that run through of a simple user-defined function in R - let us know how you get on!
If you found this useful, please share it!
For our post on user-defined functions in Python - click here!
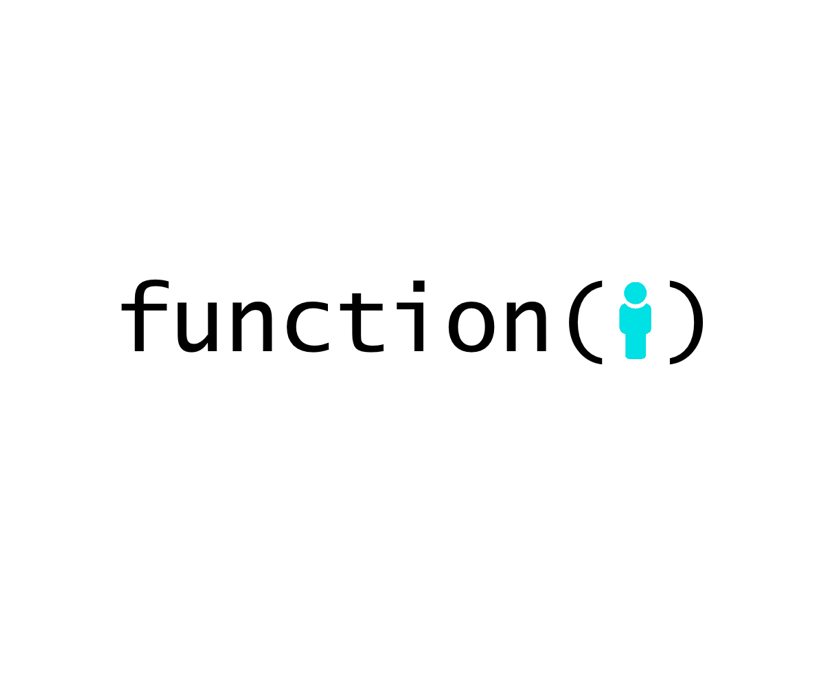
Comentários