HOW TO: R - Your first For Loop
- Andrew Jones
- Aug 1, 2017
- 2 min read
For our post on For Loop's in Python - click here!
In many programming languages, For Loops are used to repeat a specific block of code. Many programmers refer to the 'DRY' principle (Don't Repeat Yourself!) as a best practice, and this will help ensure you don't!
Imagine this simple scenario - you want to print out a list of text showing increasing page numbers in a book
You could do this manually, like below:
print(paste("The page number is", 1))
"The page number is 1"
print(paste("The page number is", 2))
"The page number is 2"
print(paste("The page number is", 3))
"The page number is 3"
print(paste("The page number is", 4))
"The page number is 4"
print(paste("The page number is", 5))
"The page number is 5"
This works fine and gives you exactly the output you wanted, but what if instead of 5 pages would wanted to print 500 pages - it now becomes very time consuming
Instead, let's make R do all the hard work by putting in place a For Loop
for (page_number in c(1,2,3,4,5)){
print(paste("The page number is", page_number))
}
"The page number is 1"
"The page number is 2"
"The page number is 3"
"The page number is 4"
"The page number is 5"
In the scenario we mentioned above, where we needed 500 pages listed, it's just as easy!
for (page_number in 1:500)){
print(paste("The page number is", page_number))
}
"The page number is 1"
"The page number is 2"
"The page number is 3"
....
....
"The page number is 499"
"The page number is 500"
Note:
You may often hear that R programmers avoid using loops where possible. This is because there are more efficient ways to process the data (essentially, in each repetition of the for loop, R has to re-size the vector and re-allocate memory. It has to find the vector in memory, create a new vector that will fit more data, copy the old data over, insert the new data, and erase the old vector. This can get very slow as vectors get larger)
However! For small to medium sized data you won't run into any genuine problems and I still think they're extremely useful to know and understand!
That was a basic run through of a For Loop in R, if it was helpful, please share!
For our post on For Loop's in Python - click here!
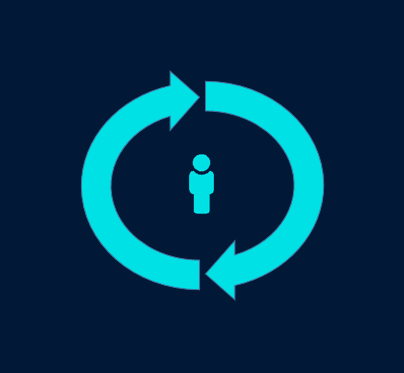
Comments